There are two plugins to get battery status using Cordova, the first one is called phonegap-plugin-battery-status , and the second one is cordova-plugin-battery-status .
Table of Contents
phonegap-plugin-battery-status
The phonegap-plugin-battery-status
plugin is deprecated , but it is still working .
It can be used to get information , such as when the battery is being charged or not : onchargingchange
, what is the charge of the battery : onlevelchange
, how much time is remaining to charge the device : onchargingtimechange
, and how much time is remaining for the device to discharge : ondischargingtimechange
. The last two information can be gotten on android only .
Create a demo application :
$ cordova create demo-pg-battery-status com.twiserandom.mobileapps.demo.pgBatteryStatus "Pg Battery Status" $ cd demo-pg-battery-status $ cordova platform add ios $ cordova platform add android $ cordova plugin add phonegap-plugin-battery-status
Edit the www/index.html
to look like this :
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta http-equiv="Content-Security-Policy" content="default-src 'self' data: gap: https://ssl.gstatic.com 'unsafe-eval'; style-src 'self' 'unsafe-inline'; media-src *; img-src 'self' data: content:;"> <meta name="format-detection" content="telephone=no"> <meta name="msapplication-tap-highlight" content="no"> <meta name="viewport" content="initial-scale=1, width=device-width, viewport-fit=cover"> <meta name="color-scheme" content="light dark"> <title>Demo Phonegap Plugin Battery Status</title> </head> <body> <div class="app"> <div id="console"> <h1>Battery Status</h1> </div> </div> <script src="cordova.js"></script> <script src="js/index.js"></script> </body> </html>
And edit the www/js/index.js
, to look like this :
document.addEventListener('deviceready', onDeviceReady, false); function onDeviceReady() { let console = document.getElementById("console"); function logStatusObject(){ console.innerHTML += `<p>level: ${this.level}</p>`; console.innerHTML += `<p>charging: ${this.charging}</p>`; console.innerHTML += `<p>chargingTime: ${this.chargingTime}</p>`; console.innerHTML += `<p>dischargingTime: ${this.dischargingTime}</p>`; console.innerHTML += "<hr/>"; } navigator .getBattery() .then( function (battery) { battery.onchargingchange = logStatusObject; battery.onlevelchange = logStatusObject; battery.onchargingtimechange = logStatusObject; battery.ondischargingtimechange = logStatusObject; }); }
Run , the application using :
$ cordova emulate android
And check the status messages gotten using the plugin .
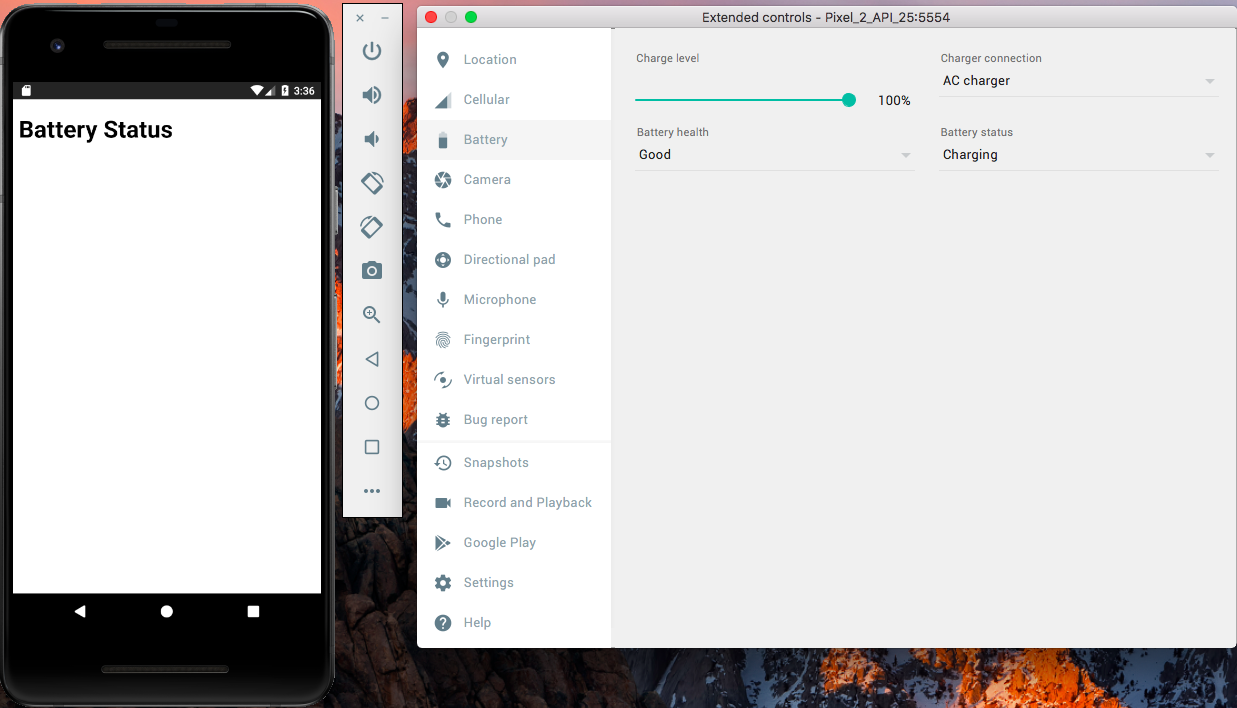
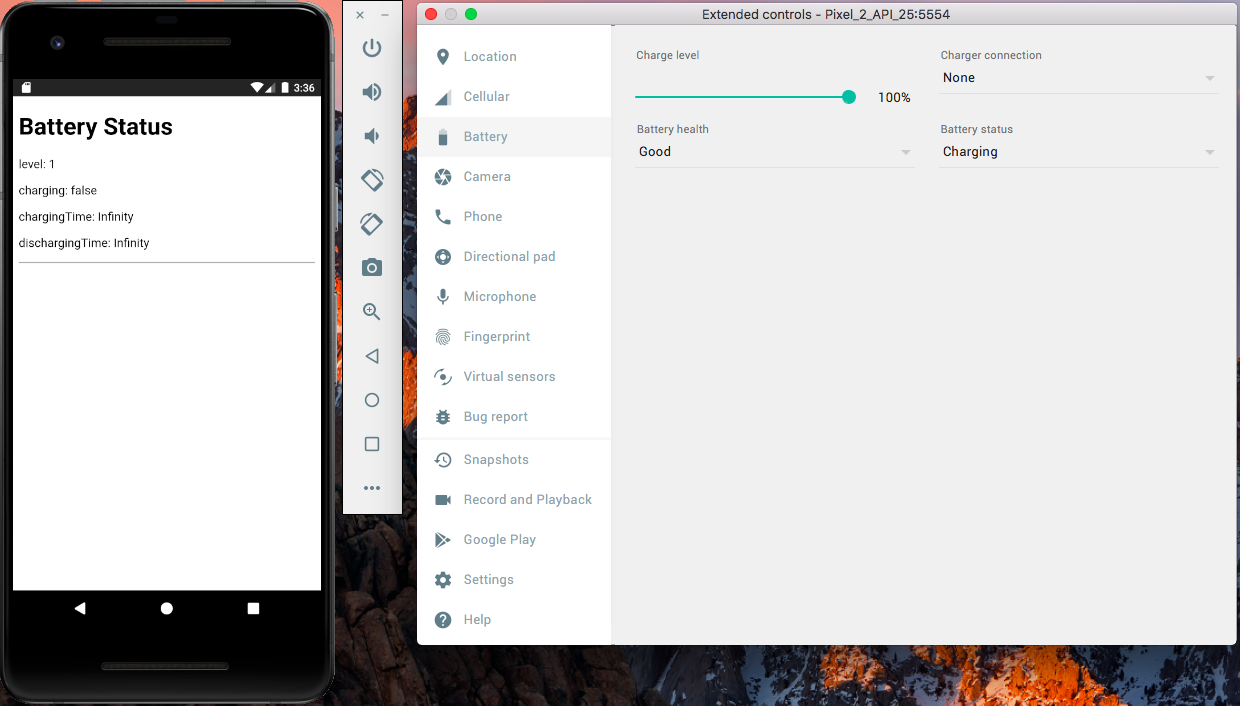
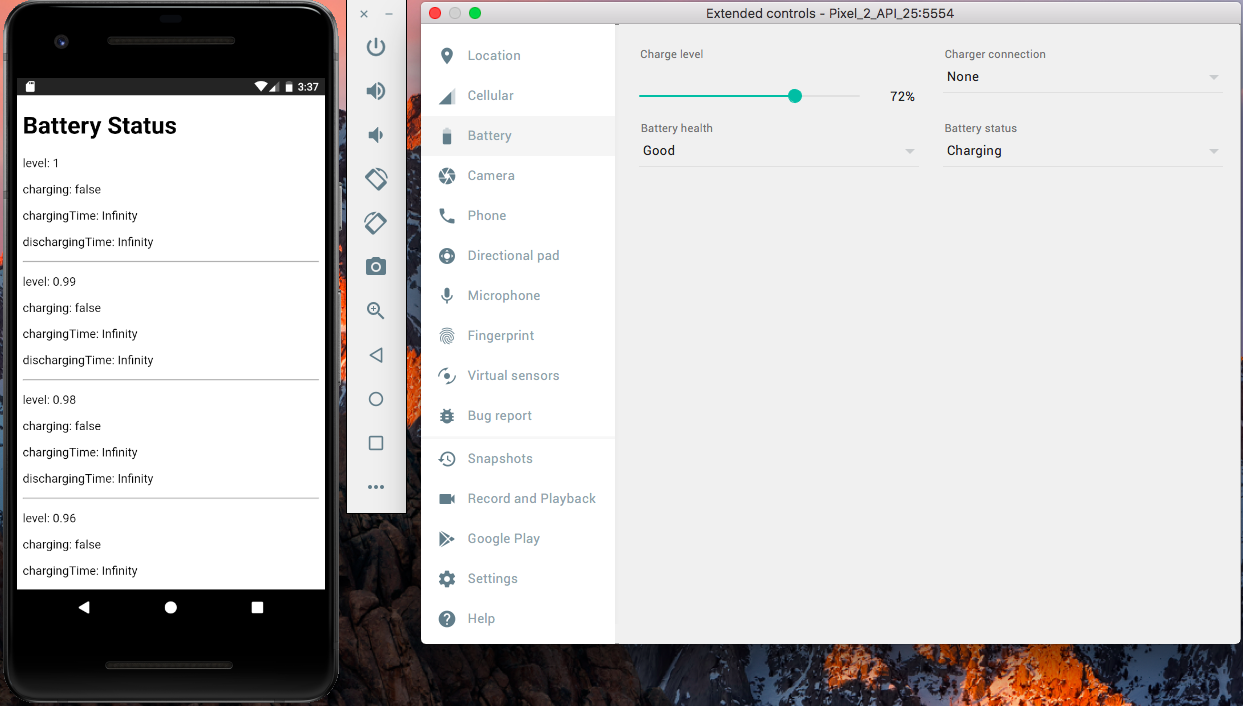
cordova-plugin-battery-status
This plugin can be used to get information about when when the battery reaches a critical level : batterycritical
, or a low level : batterylow
, and when the battery charge changes : batterystatus
, or when the phone is being plugged or unplugged : batterystatus
.
Create an application :
$ cordova create demo-cp-battery-status com.twiserandom.mobileapps.demoCpBatteryCtatus "Demo Cp Battery Status" $ cd demo-cp-battery-status $ cordova platform add ios $ cordova platform add android $ cordova plugin add cordova-plugin-battery-status
Edit www/index.html
to look like this :
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta http-equiv="Content-Security-Policy" content="default-src 'self' data: gap: https://ssl.gstatic.com 'unsafe-eval'; style-src 'self' 'unsafe-inline'; media-src *; img-src 'self' data: content:;"> <meta name="format-detection" content="telephone=no"> <meta name="msapplication-tap-highlight" content="no"> <meta name="viewport" content="initial-scale=1, width=device-width, viewport-fit=cover"> <meta name="color-scheme" content="light dark"> <title>Demo Cordova Plugin Battery Status</title> </head> <body> <div class="app"> <div id="console"> <h1>Battery Status</h1> </div> </div> <script src="cordova.js"></script> <script src="js/index.js"></script> </body> </html>
Edit www/js/index.js
to look like this :
document.addEventListener('deviceready', onDeviceReady, false); function onDeviceReady() { let console = document.getElementById("console"); function logStatusObject(status){ console.innerHTML += `<p>level: ${status.level}</p>`; console.innerHTML += `<p>isPlugged: ${status.isPlugged}</p>`; console.innerHTML += "<hr/>"; } window.addEventListener("batterystatus", logStatusObject, false); window.addEventListener("batterylow", logStatusObject, false); window.addEventListener("batterycritical", logStatusObject, false); }
Run , the application using :
$ cordova emulate android
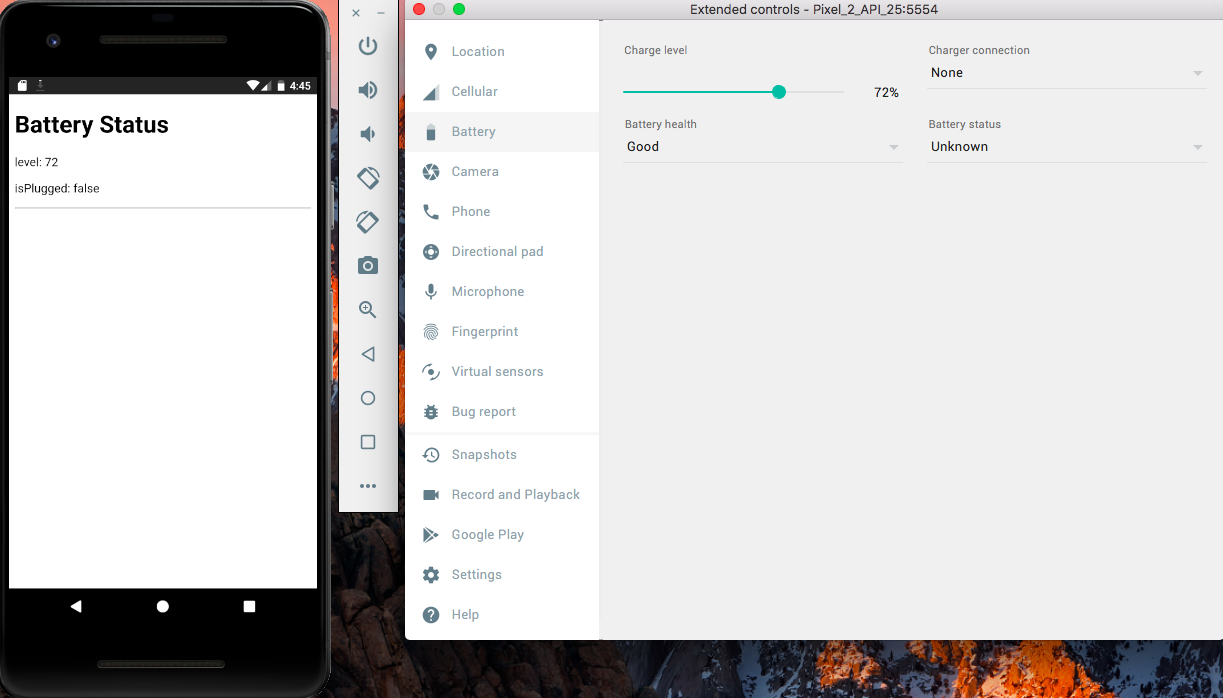
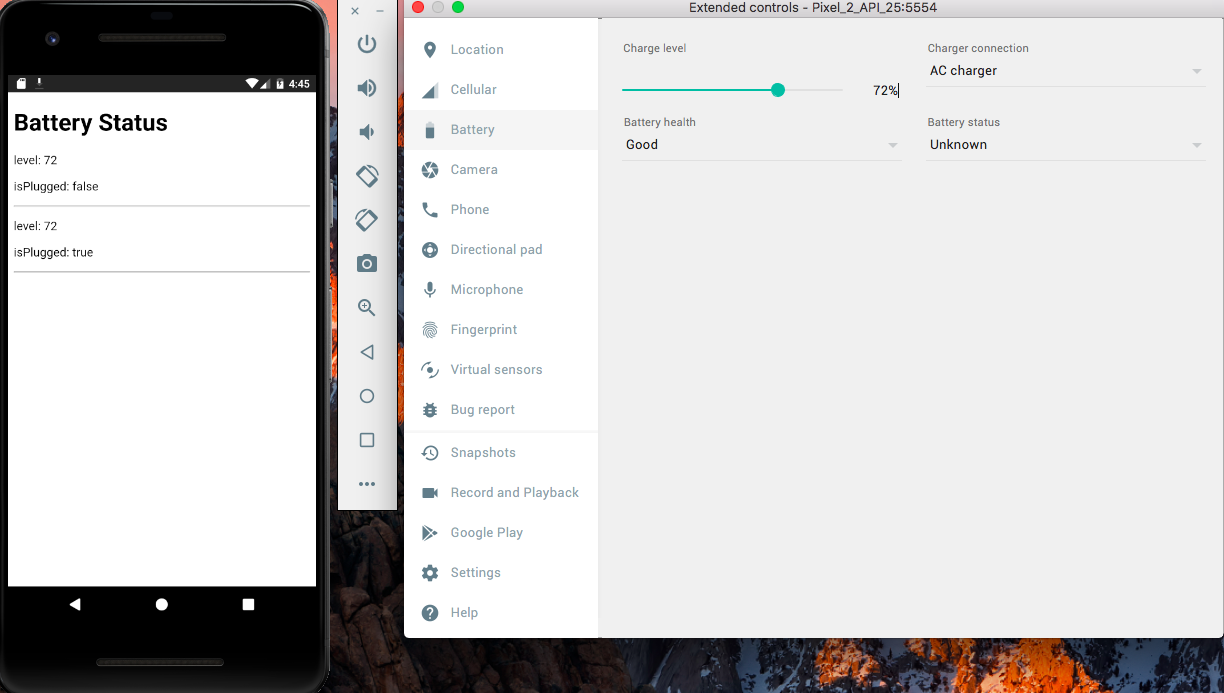
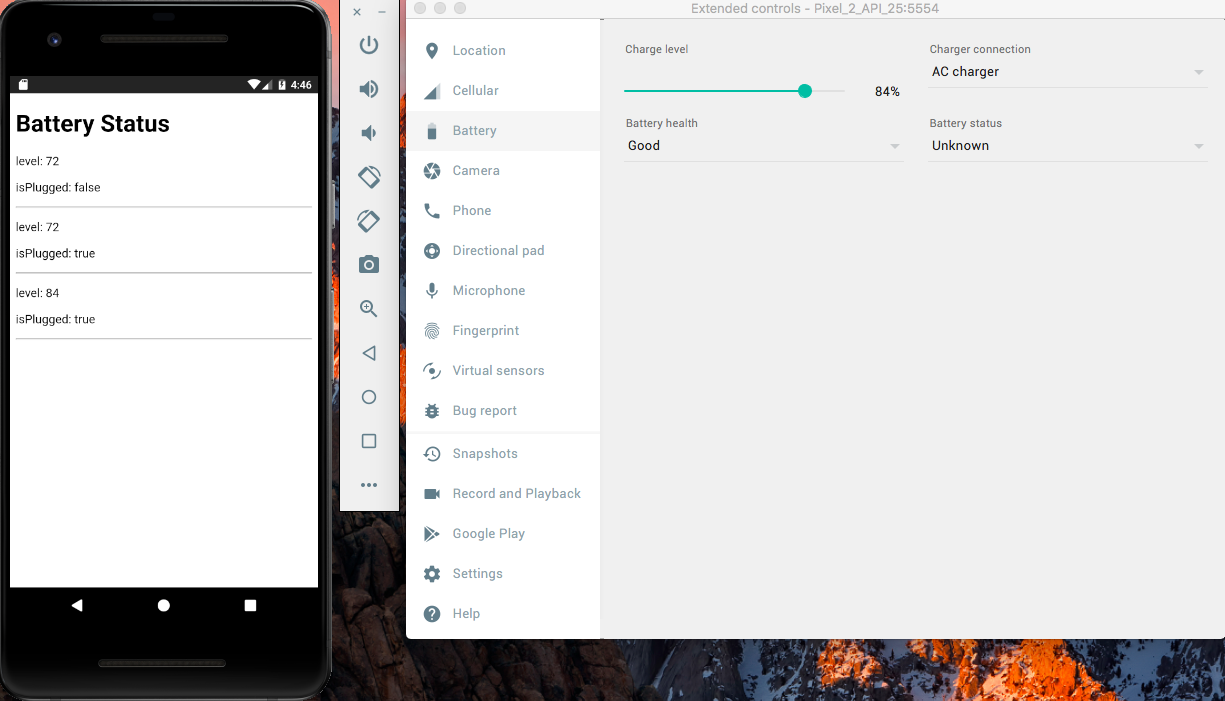
Can getting a battery reading be used to track user activity ?
Well probably , by using differential analysis , to a certain degree of certitude it can be known what the user is doing .
Research is made , on how much battery a phone will consume , based on what the user is doing . Like for example , if he is watching movies , then the rate of battery consumption is such , if he’s listening to music , then the rate of battery consumption is such , if he is using social media , then it is such …
Given research is made , it can be analyzed based on the rate of change in the battery level , when the phone is being plugged or unplugged , to construct with a certain degree of knowledge , what the user has been doing .